集合
含义
容器,位于java.util包下面的类
数组特点
- 大小固定[不会自动扩容]
- 可以存基本数据、引用数据类型
集合特点
示意图
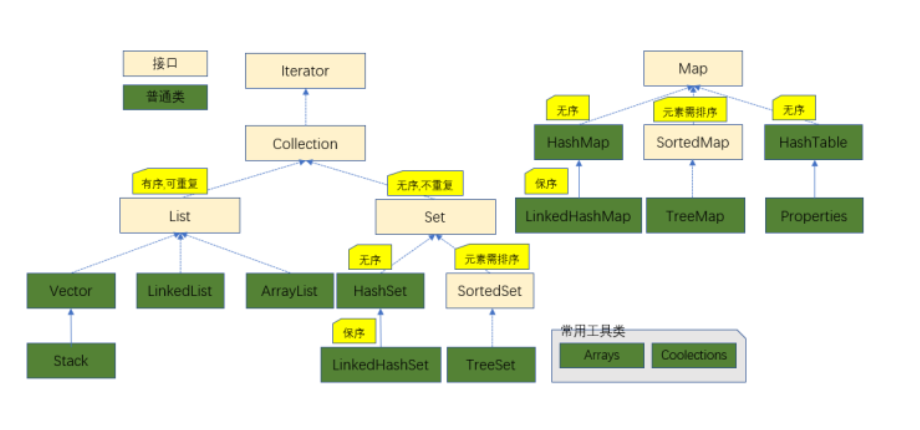
ArrayList
含义
是动态变长的数组
底层
是动态变长的数组
体系结构
常用方法
- isEmpty
- size
- add
- remove
- set
- contains
- get
- clear
- toArray
- addAll 将集合参数的元素逐个加入
- removeAll 将集合参数中的元素中出现的逐个删除
- containsAll 判断集合参数中的元素释放全部都存在
- indexOf 获取元素第一次出现的位置
- lastIndexOf 获取元素最后一次出现的位置
- 等等
泛型
含义
参数化类型(将操作的数据类型放到<>中)
好处
使用场景
案例一
Animal.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class Animal<T> { private T content; public void print(T content){ this.content = content; System.out.println(content); } public void say(){ System.out.println(content); } public T getContent(){ return content; } }
|
GenericDemo.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public class GenericDemo { public static void main(String[] args) { Animal<String> animal = new Animal<String>(); animal.print("11"); animal.print("sdfsdf"); Animal<Integer> other = new Animal<Integer>(); other.print(1); Integer xx = other.getContent(); }
}
|
案例二
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class _02GenericDemo { public static void main(String[] args) { Integer result = getData(true); System.out.println(result); String otherReuslt =getData(false); System.out.println(otherReuslt); String data = getData("xxx"); } public static <T> T getData(boolean sign){ if(sign == true){ return (T) new Integer(11); }else{ return (T) "xx"; } } public static <T> T getData(T content){ return content; } }
|
案例三
User.java
Student.java
1 2 3
| public class Student extends User {
}
|
_03GenericDemo.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| public class _03GenericDemo { public static void main(String[] args) { List<Integer> data = new ArrayList<Integer>(); data.add(11); int result = data.get(0); System.out.println(result); List<String> otherData = new ArrayList<String>(); add(data); add(otherData); List<User> userData = new ArrayList<User>(); List<Student> studentData = new ArrayList<Student>(); append(userData); append(studentData); insert(userData); insert(studentData); } public static void add(List<?> data){ } public static void append(List<? extends User> data){ } public static void insert(List<? super Student> data){ } }
|
Vector
含义
线程安全的ArrayList,使用是上和ArrayList使用差不多
vs ArrayList
- 都实现List接口
- 底层都是采用可变数组存储
- vector是线程安全的
体系结构
常用方法
- add addAll
- remove removeAll
- contains containsAll
- set
- get
- isEmpty
- size
- indexOf lastIndexOf
- toArray
- clear
- 等等
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| public class VectorDemo { public static void main(String[] args) { Vector<String> data = new Vector<>(); data.add("11"); System.out.println(data.get(0)); } }
|
Stack
含义
栈数据结构
体系
底层
本质采用可变数组
常用方法
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| public class StackDemo { public static void main(String[] args) { Stack<String> stack = new Stack<>(); stack.push("11"); stack.push("22"); stack.push("33"); System.out.println(stack.peek()); System.out.println(stack.pop()); System.out.println(stack.empty()); System.out.println(stack); } }
|
LinkedList
含义
JDK提供的类,该类实现双向链表数据结构
示意图

底层
双向链表
体系结构
常用方法
和ArrayList差不多
案例一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| public class _01LinkedListDemo { public static void main(String[] args) { LinkedList<String> data = new LinkedList<String>(); data.add("11"); data.add("22"); data.add("33"); System.out.println(data.get(0)); } }
|
案例二
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| public class _02Compare { public static void main(String[] args) { add(100000); } public static void add(int max){ long start,end; List<Integer> arrayList = new ArrayList<>(); start = System.currentTimeMillis(); for(int i=0;i<max;i++){ arrayList.add(0,i); } end = System.currentTimeMillis(); System.out.println("arrayList的插入时间:"+(end-start)); List<Integer> linkedList = new LinkedList<>(); start = System.currentTimeMillis(); for(int i=0;i<max;i++){ linkedList.add(0,i); } end = System.currentTimeMillis(); System.out.println("linkedList的插入时间:"+(end-start)); }
}
|
vs ArrayList
|
ArrayList |
LinkedList |
数据结构 |
数组 |
链表 |
是否有索引 |
有 |
没有 |
根据索引查询 |
快 |
慢(只能遍历后比较位置) |
更新 |
快(通过索引快速找到更新) |
慢(只能遍历找到后更新) |
删除 |
慢(需要移动位置) |
快(不需要移动位置) |
添加 |
慢(需要移动位置) |
快(不需要移动位置) |
单向队列[了解]
特点
FIFO\LILO
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| public class QueueDemo { public static void main(String[] args) { Queue<Integer> queue = new LinkedList<>(); queue.add(1); queue.offer(2); queue.offer(3); System.out.println(queue); queue.remove(); System.out.println(queue); queue.poll(); System.out.println(queue); int result = queue.element(); result = queue.peek(); System.out.println(result); } }
|
双向队列[了解]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class DequeDemo { public static void main(String[] args) { Deque<Integer> data = new LinkedList<>(); } }
|
HashMap 示意代码
MyHashMap.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62
| public class MyHashMap<K,V> { private final int INIT_CAPACITY=1000; private Node<K,V> [] container = new Node[INIT_CAPACITY]; public MyHashMap(){ } public void put(K key,V value){ int code = key.hashCode()>>2; Node<K,V> oldNode = container[code]; if(oldNode == null){ Node<K,V> node = new Node<K,V>(); node.hashCode = code; node.key=key; node.value = value; container[code] = node; }else{ K oldKey = oldNode.key; if(oldKey.equals(key)){ oldNode.value=value; }else{ Node<K,V> node = new Node<K,V>(); node.hashCode = code; node.key=key; node.value = value; oldNode.next=node; } } } public Object get(K key){ int code = (key.hashCode()>>2); return container[code].value; } }
class Node<K,V>{ int hashCode; K key; V value; Node<K,V> next; }
|
Test.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| package com.neu.day08._06map;
public class Test { public static void main(String[] args) { MyHashMap<User, String> data = new MyHashMap<>(); User one = new User(100); data.put(one, "xx"); User two = new User(100); data.put(two, "yy"); }
}
class User{ int num;
public User(int num){ this.num = num; } @Override public int hashCode() { final int prime = 31; int result = 1; result = prime * result + num; return result; } }
|
HashMap
含义
key-value形式
底层
数组+链表
示意图
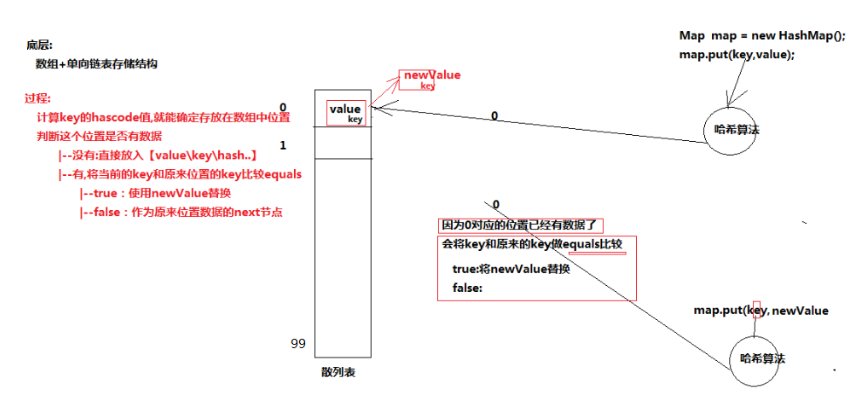
体系结构
常用方法
- size
- put
- putAll
- get
- size
- isEmpty
- clear
- containsKey
- containsValue
- remove
- 等等
案例一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| public class _01HashMapDemo { public static void main(String[] args) { HashMap<String,String> data = new HashMap<>(); data.put("name","zs"); data.put("age","10"); data.put("address","guangzhou"); System.out.println(data.get("name")); data.remove("age"); System.out.println(data); System.out.println(data.containsKey("name")); System.out.println(data.containsValue("zs")); System.out.println(data); Map<String,String> temp = new HashMap<>(); temp.put("color", "red"); temp.put("size", "xl"); data.putAll(temp); System.out.println(data); System.out.println(data.size()); } }
|
案例二
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| public class _02HashMapDemo { public static void main(String[] args) { HashMap<String,String> data = new HashMap<>(); data.put("name","zs"); data.put("age","10"); data.put("address","guangzhou"); System.out.println("方式一................"); Set<String> keys = data.keySet(); for(String key : keys){ System.out.println(key+"="+data.get(key)); } System.out.println("方式二................"); Collection<String> values = data.values(); for(String value : values){ System.out.println(value); } System.out.println("方式三................"); Set<Entry<String, String>> entrySet = data.entrySet(); for(Entry<String, String> entry : entrySet){ System.out.println(entry.getKey()+"="+entry.getValue()); } } }
|
案例三
验证HashMap是否有序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class _03HashMapDemo { public static void main(String[] args) { Map<String,String> data = new HashMap<>(); data.put("33","33"); data.put("hhhhhh","33"); data.put("aa","aaaa"); data.put("xx","xxxx"); data.put("yy","yyyy"); data.put("yy","1000"); data.put("11","11"); data.put("22","22"); System.out.println(data); } }
|
LinkedHashMap
含义
有序的hashMap
体系结构
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class _04LinkedHashMap { public static void main(String[] args) {
Map<String,String> data = new LinkedHashMap<>(); data.put("33","33"); data.put("hhhhhh","33"); data.put("aa","aaaa"); data.put("xx","xxxx"); data.put("yy","yyyy"); data.put("yy","1000"); data.put("11","11"); data.put("22","22"); System.out.println(data); } }
|
Hashtable
含义
重量级HashMap,用法和HashMap类似
体系结构
和HashMap的区别
- Hashtable的方法几乎都有synchronized【线程安全】
- HashMap的key和value都是可以为null,Hashtable的key和value都不可以为空
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class _05HashtableDemo { public static void main(String[] args) { Hashtable<String,String> data = new Hashtable<>(); data.put("xx", "xxxx"); data.put("yy", "yyyy"); data.put("zz", "zzzz"); System.out.println(data); HashMap<String,String> xx = new HashMap<>(); xx.put(null,null); } }
|