File
含义
File对象表示一个文件或文件夹
常用方法
pathSeparator
pathSeparatorChar
separator
separatorChar
new File(String pathName)
new File(String parentName,String childName)
new File(File parentFile,String childName)
mkdir
mkdirs
createNewFile
delete
删除普通文件或空目录
renameTo
exists
isFile
isDirectory
getParent
getParentFile
getAbsolutePath
list
listFiles
案例一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class _01FileDemo { public static void main(String[] args) { System.out.println(File.pathSeparator); System.out.println(File.pathSeparatorChar); System.out.println(File.separator); System.out.println(File.separatorChar); File file1 = new File("C:\\abc.txt"); System.out.println(file1.isFile()); File file2 = new File("C:", "abc.txt"); System.out.println(file2.isDirectory()); File file3 = new File(new File("C:"), "abc"); System.out.println(file3.exists()); } }
|
案例二
创建、删除
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| public class _02FileDemo { public static void main(String[] args) throws IOException { File file = new File("C:\\efg"); System.out.println(file.exists()); boolean sign = false; if(!file.exists()){ sign = file.mkdir(); System.out.println(sign); } File otherFile = new File("C:\\11\\22\\33"); sign = otherFile.mkdirs(); System.out.println(sign); new File("C:\\abc.txt").delete(); new File("C:\\efg").delete(); new File("C:\\11").delete(); file = new File("C:\\11.txt"); if(!file.exists()){ file.createNewFile(); } file.renameTo(new File("C:\\22.txt")); } }
|
案例三
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public class _03FileDemo { public static void main(String[] args) throws IOException { File file = new File("C:\\22.txt"); System.out.println(file.isFile()); System.out.println(file.isDirectory()); System.out.println(file.exists()); System.out.println(file.getParent()); System.out.println(file.getParentFile()); System.out.println(file.isAbsolute()); System.out.println(file.getAbsolutePath()); } }
|
案例四
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| public class _04FileDemo { public static void main(String[] args) throws IOException { File file = new File("D:\\java6\\02javase\\Day10"); System.out.println(Arrays.toString(file.list())); File [] children = file.listFiles(); for(File child:children){ System.out.println(child.getAbsolutePath()); } System.out.println("过滤........."); children = file.listFiles(new FileFilter() { @Override public boolean accept(File pathname) { return pathname.getName().endsWith(".txt"); } }); for(File child:children){ System.out.println(child.getAbsolutePath()); } } }
|
案例五
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class _05FileDemo { public static void main(String[] args) throws IOException { listFileName("D:\\java6\\02javase\\Day09","|"); } public static void listFileName(String path,String prefix){ File file = new File(path); if(file.isFile()){ System.out.println(file.getName()); }else{ //逻辑孩子 File childrent [] = file.listFiles(); for(File child:childrent){ if(child.isFile()){ System.out.println(prefix+child.getName()); }else{ System.err.println(prefix+child.getName()); //又是一个文件夹 listFileName(child.getAbsolutePath(),prefix+"-"); } } } } }
|
IO
含义
输入输出流
分类
使用场景
字节流
含义
传输单位一个一个字节
使用场景
操作非文本文件
mp3、mp4、压缩文件等等
分类
字节流输入流
体系结构
常用方法
案例一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| public class FileInputStreamDemo { public static void main(String[] args) { readFile("C:\\abc.txt"); } public static void readFile(String path){ InputStream inputStream=null; try { inputStream = new FileInputStream(path); StringBuilder SB = new StringBuilder(); int result = -1; while((result=inputStream.read())!=-1){ SB.append((char)result); } System.out.println(SB); } catch (IOException e) { e.printStackTrace(); }finally { if(inputStream!=null){ try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } }
}
|
案例二
使用缓冲
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public class FileInputStreamDemoOther { public static void main(String[] args) { String result = readFile("C:\\abc.txt"); System.out.println(result); } public static String readFile(String path){ StringBuilder SB = null; InputStream inputStream=null; try { inputStream = new FileInputStream("C:\\abc.txt"); SB = new StringBuilder(); byte [] buffer = new byte[3]; int len = -1; while((len = inputStream.read(buffer))!=-1){ SB.append(new String(buffer,0,len)); } return SB.toString(); } catch (IOException e) { e.printStackTrace(); return null; }finally { if(inputStream!=null){ try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
|
字节输入缓冲流
含义
字节缓冲输入流
常用方法
示意图
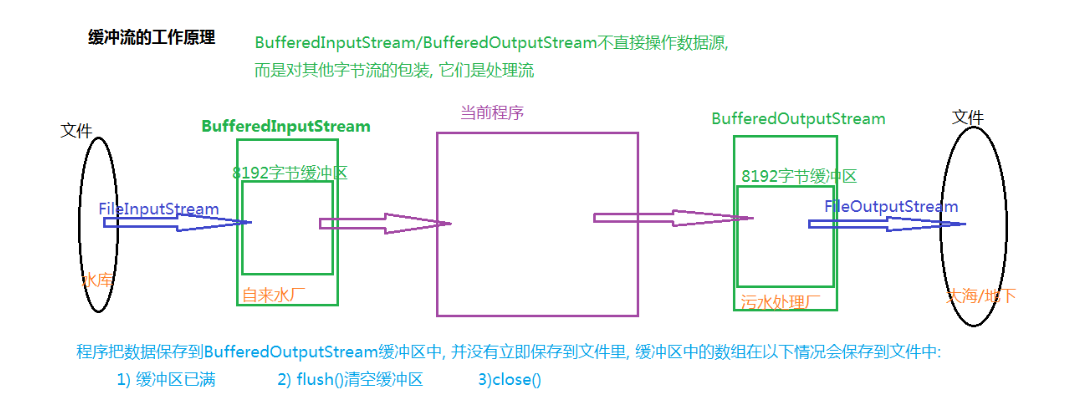
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| public class BufferedInputSteamDemo { public static void main(String[] args) { String path = "C:\\abc.txt"; readFile(path); } public static void readFile(String path){ InputStream inputStream = null; BufferedInputStream bufferedInputStream =null; try { inputStream = new FileInputStream(path); bufferedInputStream = new BufferedInputStream(inputStream); byte [] cache = new byte[1024]; int len = -1; while((len=bufferedInputStream.read(cache))!=-1){ System.out.println(new String(cache,0,len)); } } catch (IOException e) { e.printStackTrace(); }finally { if(bufferedInputStream!=null){ try { bufferedInputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
|
字节输出流
含义
字节输出流
体系结构
常用方法
- new FileOutputStream(Strint fileName)
- new FileOutputStream(File file)
- write
- close
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| public class FileOutputStreamDemo { public static void main(String[] args) { String des = "C:\\xx\\33.txt"; writeToFile("中国加油",des); } public static void writeToFile(String content,String dest){ File target = new File(dest); if(!target.getParentFile().exists()){ target.getParentFile().mkdirs(); } OutputStream outputStream=null; try { outputStream = new FileOutputStream(target); outputStream.write(content.getBytes()); } catch (IOException e) { e.printStackTrace(); }finally { if(outputStream!=null){ try { outputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
|
字节输出缓冲流
含义
对字节输出流的包装,内置有缓冲区。
常用方法
write
flush
将缓冲区的数据输出到目标中
close
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public class BufferedOutputSteamDemo { public static void main(String[] args) { writeFile("JAVA","c:\\yy\\aa.txt"); } public static void writeFile(String content,String target){ File targetFile = new File(target); if(!targetFile.getParentFile().exists()){ targetFile.getParentFile().mkdirs(); } BufferedOutputStream bufferedOutputStream=null; try { bufferedOutputStream = new BufferedOutputStream(new FileOutputStream(target)); bufferedOutputStream.write(content.getBytes()); bufferedOutputStream.flush(); } catch (IOException e) { e.printStackTrace(); }finally { if(bufferedOutputStream!=null){ try { bufferedOutputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
|
字节流文件复制
思路
一边读取一边输出
代码
StringUtil.java
1 2 3 4 5 6
| public class StringUtil { public static boolean isEmpty(String param){ return param == null || "".equals(param.trim()); } }
|
ParamException.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class ParamException extends RuntimeException { public ParamException() { super(); }
public ParamException(String message) { super(message); }
public ParamException(String message, Throwable cause) { super(message, cause); }
public ParamException(Throwable cause) { super(cause); } }
|
FileUtil.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
|
public class FileUtil { public static void main(String[] args) { boolean result = copyFile("C:\\abc.txt","D:\\xx\\yy\\22.txt"); System.out.println(result); }
public static boolean copyFile(String source,String target){ if(StringUtil.isEmpty(source) || StringUtil.isEmpty(target)){ throw new ParamException("参数都不能为空"); } if(!new File(source).exists()){ throw new ParamException("参数错误"); } File targetFile = new File(target); if(!targetFile.getParentFile().exists()){ targetFile.getParentFile().mkdirs(); } BufferedInputStream bufferedInputStream=null; BufferedOutputStream bufferedOutputStream=null; try { bufferedInputStream = new BufferedInputStream(new FileInputStream(source)); bufferedOutputStream = new BufferedOutputStream(new FileOutputStream(target)); byte [] cache = new byte[1024]; int len = -1; while((len=bufferedInputStream.read(cache))!=-1){ bufferedOutputStream.write(cache, 0, len); bufferedOutputStream.flush(); } return true; } catch (IOException e) { e.printStackTrace(); }finally { if(bufferedInputStream!=null){ try { bufferedInputStream.close(); } catch (IOException e) { e.printStackTrace(); } } if(bufferedOutputStream!=null){ try { bufferedOutputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } return false; } }
|
字符流
含义
传输单位一个一个字符
使用场景
操作文本文件
txt\xml\html\properties等等
分类
字符输入流
含义
字符输入流
体系结构
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
| public class _01FileReadDemo { public static void main(String[] args) { String source = "C:\\aa.txt"; String result = readFile(source); System.out.println(result); } public static String readFile(String source) {
Reader reader=null; try { reader = new FileReader(source); StringBuilder SB = new StringBuilder(); char [] buffer = new char[1024]; int len = -1; while((len=reader.read(buffer))!=-1){ SB.append(new String(buffer,0,len)); } return SB.toString(); } catch (IOException e) { e.printStackTrace(); }finally { if(reader!=null){ try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } return null; } }
|
字符输出流
含义
字符输出流
体系结构
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| public class _02FileWriterDemo { public static void main(String[] args) { boolean sign = writeFile("广州你好\r\n深圳你好","C:\\result.txt"); System.out.println(sign); } public static boolean writeFile(String content,String target) { Writer writer=null; try { writer = new FileWriter(target); writer.write(content); return true; } catch (IOException e) { e.printStackTrace(); }finally { if(writer!=null){ try { writer.close(); } catch (IOException e) { e.printStackTrace(); } } } return false; } }
|
字符流文件复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| public class _03FileCopy { public static void main(String[] args) { copyFile("C:\\aa.txt","D:\\result.txt"); } private static boolean copyFile(String source,String target) { BufferedReader bufferedReader=null; BufferedWriter bufferedWriter=null; try { bufferedReader = new BufferedReader(new FileReader(source)); bufferedWriter = new BufferedWriter(new FileWriter(target)); String content = null; while((content = bufferedReader.readLine())!=null){ bufferedWriter.write(content); bufferedWriter.newLine(); } return true; } catch (IOException e) { e.printStackTrace(); }finally { if(bufferedReader!=null){ try { bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } if(bufferedWriter!=null){ try { bufferedWriter.close(); } catch (IOException e) { e.printStackTrace(); } } } return false; } }
|
代码行数统计
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
| public class Test {
private static int count;
public static void main(String[] args) {
listJava("C:\\Users\\Administrator\\workspace"); System.out.println(count); }
private static void listJava(String path) {
File file = new File(path);
File[] children = file.listFiles(new FileFilter() {
@Override public boolean accept(File file) { if (file.isDirectory()) { return true; } else { return file.getName().endsWith(".java"); } } }); for (File child : children) { if (child.isFile()) { count +=lineCount(child.getAbsolutePath()); } else { listJava(child.getAbsolutePath()); } }
} private static int lineCount(String path){ int count=0; BufferedReader reader = null; try { reader = new BufferedReader(new FileReader(path)); String content = null; while((content = reader.readLine())!=null){ if(!"".equals(content.trim())){ count++; } } } catch (IOException e) { e.printStackTrace(); }finally { if(reader!=null){ try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } return count; } }
|
文件下载
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| package com.neu.day10._04download;
import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL;
public class DownloadDemo { public static void main(String[] args) { try { boolean sign = download("https://www.jxycu.edu.cn/","C:\\index.html"); System.out.println(sign); } catch (MalformedURLException e) { e.printStackTrace(); } } public static boolean download(String source,String target) throws MalformedURLException{ URL url = new URL(source); HttpURLConnection connection =null; OutputStream outputStream=null; try { connection = (HttpURLConnection) url.openConnection(); InputStream inputStream = connection.getInputStream(); outputStream = new FileOutputStream(target); int len = -1; byte [] cache = new byte[1024]; while((len=inputStream.read(cache))!=-1){ outputStream.write(cache, 0, len); } return true; } catch (IOException e) { e.printStackTrace(); }finally { connection.disconnect(); } return false; } }
|
Properties
含义
加载配置文件的
体系结构
作用
读取配置文件其实就是properties文件
避免硬编码
硬编码意思是将软件的配置信息写死在java代码中,修改麻烦。
使用步骤
在资源文件夹下新建xx.properties文件
建议:新建名字为config的资源文件夹
往里面添加数据
key1=value2
keyN=valueN
通过IO读取该配置文件中的内容
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| public class JDBCUtil { private static String name; private static String password; static{ Properties properties = new Properties(); ClassLoader classLoader = JDBCUtil.class.getClassLoader(); InputStream inputStream = null; try { inputStream = classLoader.getResourceAsStream("jdbc.properties"); properties.load(inputStream); name = properties.getProperty("name"); password = properties.getProperty("password"); } catch (IOException e) { e.printStackTrace(); }finally { if(inputStream!=null){ try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } public static String getName() { return name; }
public static String getPassword() { return password; } }
|