String 补充
转换示意图
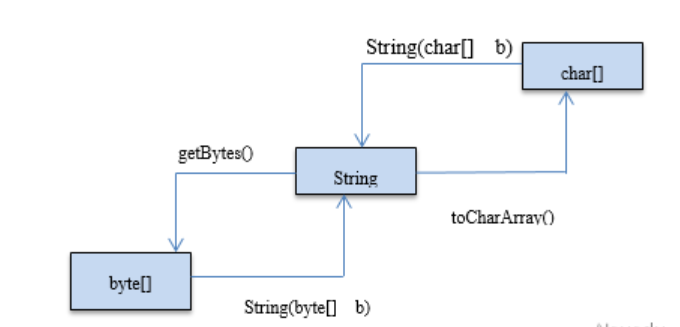
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class StringDemo { public static void main(String[] args) { String name = "abc"; char[] values = name.toCharArray(); System.out.println(Arrays.toString(values)); String otherName = new String(values); System.out.println(otherName); otherName = new String(values, 1, 2); System.out.println(otherName); System.out.println(name); byte [] bytes = name.getBytes(); System.out.println(Arrays.toString(bytes)); otherName = new String(bytes, 0, 2); System.out.println(otherName); }
}
|
StringBuilder
含义
可变字符串
使用场景
做字符串拼接等操作
常用方法
- new StringBuilder()
- new StringBuilder(CharSequence data)
- append
- insert
- deleteCharAt
- reverse
- 等等大量和String差不多
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| public class StringBuilderDemo { public static void main(String[] args) { String name = "11"; System.out.println(name.hashCode()); name = name + "22"; System.out.println(name.hashCode()); StringBuilder SB = new StringBuilder();
System.out.println(SB.hashCode()); SB.append("11").append("22").append("33"); System.out.println(SB.toString()); System.out.println(SB.hashCode()); SB.deleteCharAt(0); System.out.println(SB); SB.insert(0,"1"); System.out.println(SB); SB.reverse(); System.out.println(SB); byte [] xx = {97,98,99}; StringBuilder data = new StringBuilder(new String(xx)); data.reverse(); xx = data.toString().getBytes(); System.out.println(Arrays.toString(xx)); } }
|
StringBuffer
含义
线程安全的可变字符串
特点
- 方法几乎都加了synchronized,代表多线程环境下是线程安全的
- 其他方法几乎和StringBuilder一样
案例
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class StringBufferDemo { public static void main(String[] args) { StringBuffer SB = new StringBuffer("abc"); SB.append("efg"); System.out.println(SB); } }
|
Date
含义
日期类
常用方法
- new Date()
- after
- before
- compareTo
- getTime
- setTime
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public class DateDemo { public static void main(String[] args) { Date dateOne = new Date(); System.out.println(dateOne.getTime()); Date dateTwo = new Date(dateOne.getTime()-1000000); System.out.println(dateOne.after(dateTwo)); System.out.println(dateOne.before(dateTwo)); System.out.println(dateOne.compareTo(dateTwo)); System.out.println(dateOne); yy(); } @Deprecated private static void yy(){ } }
|
Calendar
含义
日期的操作类
常用方法
- Calendar.getInstance()
- getTime
- setTime
- before
- after
- compareTo
- add(int field,int value)
- get(int field)
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| package com.neu.day07._04date;
import java.util.Calendar; import java.util.Date;
public class CalendarDemo {
public static void main(String[] args) { Calendar calendar = Calendar.getInstance(); Date curDate = calendar.getTime();
System.out.println(calendar.get(Calendar.YEAR)); System.out.println(calendar.get(Calendar.MONTH)+1); System.out.println(calendar.get(Calendar.DAY_OF_MONTH)); System.out.println(calendar.get(Calendar.DAY_OF_YEAR)); int week = calendar.get(Calendar.DAY_OF_WEEK); System.out.println(week); switch (week) { case Calendar.SATURDAY: break; case Calendar.THURSDAY: break;
default: break; } calendar.add(Calendar.HOUR,-1); System.out.println(calendar.getTime()); } }
|
含义
日期的格式化类
常用方法
- applyPattern
- format
- parse
常见的格式
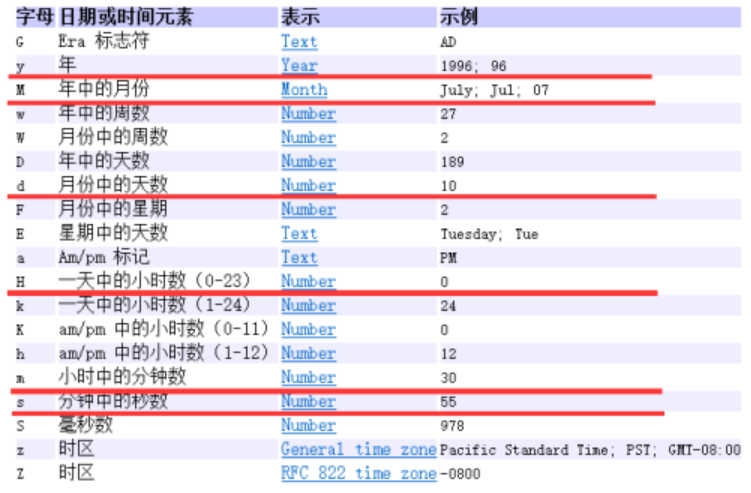
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| public class SimpleDateFormateDemo { public static void main(String[] args) { Date date= new Date(); System.out.println(date);
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); String result = simpleDateFormat.format(date); System.out.println(result); SimpleDateFormat otherFormat = new SimpleDateFormat("yyyy年MM月dd日"); try { Date otherDate = otherFormat.parse(result); System.out.println(simpleDateFormat.format(otherDate)); } catch (ParseException e) { e.printStackTrace(); } } }
|
UUID
含义
随机数
使用场景
数据库主键(char(32))
案例
1 2 3 4 5 6 7 8 9 10
| public class UUIDDemo { public static void main(String[] args) { String ID = UUID.randomUUID().toString(); System.out.println(ID.length()); System.out.println(ID); System.out.println(ID.replace("-","")); System.out.println(ID.replace("-","").length()); } }
|
享元设计模式
含义
将那些经常使用的对象缓存起来,使用的时候直接从缓存中获取
目的
避免反复创建那些经常使用的对象
步骤
- 定义一个缓存容器[比如:数组]
- 静态代码块中创建那些经常使用的对象并放入缓存容器中
- 对外提供从容器中获取对象的方法
案例
User.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| public class User { private static User [] cache = new User[3]; static{ User zs = new User("zs"); User ls = new User("ls"); User ww = new User("ww"); cache[0]=zs; cache[1]=ls; cache[2]=ww; } public static User valueOf(String name){ User result = null; for(User user : cache){ if(user.name.equals(name)){ result = user; break; } } if(result == null){ result = new User(name); } return result; } private String name; public User(String name){ this.name = name; }
@Override public String toString() { return "User [name=" + name + "]"; } }
|
Test.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class Test { public static void main(String[] args) { User userOne = User.valueOf("zs"); User userTwo = User.valueOf("zs"); System.out.println(userOne == userTwo); User userThree = User.valueOf("xx"); User userFour = User.valueOf("xx"); System.out.println(userThree == userFour); } }
|
包装数据类型
含义
8大基本数据类型都有对应的引用数据类型,这些引用数据类型一般称为包装数据类型
为什么需要有
- 包装数据类型可以表示某些特定的状态
- 包装数据类型提供了一些好用的方法
对应关系
基本数据类型 |
包装数据类型 |
byte |
Byte |
short |
Short |
char |
Character |
int |
Integer |
long |
Long |
float |
Float |
double |
Double |
boolean |
Boolean |
装箱
自行不全
拆箱
自行补全
常用方法
- intValue
- valueOf
- parseInt
- toBinaryString
- toHexString
- max
- min
- compareTo
- MAX_VALUE
- MIN_VALUE
- SIZE
案例一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84
| package com.neu.day07._07wrapper;
public class IntegerDemo { public static void main(String[] args) { Integer data = Integer.valueOf(10); int dataOther = data.intValue(); Integer num = 20; int numOther = num; System.out.println(Integer.parseInt("111")); System.out.println(Integer.toBinaryString(140)); System.out.println(Integer.MAX_VALUE); System.out.println(Integer.MIN_VALUE); System.out.println(Integer.SIZE/8); Integer one = 110; Integer two = 120; System.out.println(one > two); System.out.println(one.compareTo(two)); System.out.println(Integer.toHexString(12)); System.out.println(Integer.min(one, two)); System.out.println(Integer.max(one, two)); Integer temp = new Integer(20); } }
|
案例二
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class IntegerDemoOther { public static void main(String[] args) { Integer data1 = 1; Integer data2 = 1; System.out.println(data1==data2); Integer data3 = 127; Integer data4 = 127; System.out.println(data3==data4); Integer data5 = 128; Integer data6 = 128; System.out.println(data5==data6); Integer data7 = new Integer(1); Integer data8 = new Integer(1); System.out.println(data7==data8); } }
|
案例三
1 2 3
| 总结发现byte\short\int\long对应的包装数据类型缓存范围是:[-128,127] 总结发现char对应的包装数据类型缓存范围是:[0,127] 总结发现boolean对应的包装数据类型缓存的是:true和false
|
Sysetem[了解]
含义
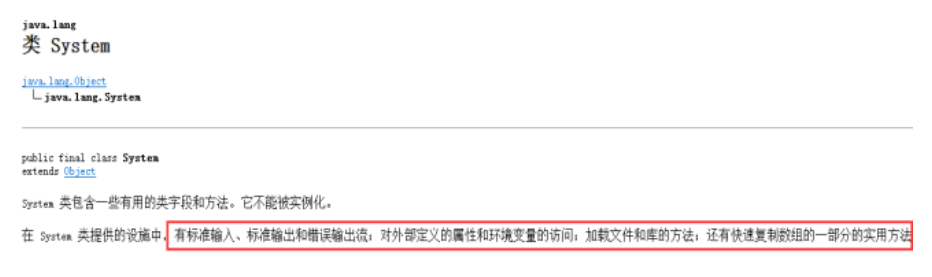
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public class SystemDemo { public static void main(String[] args) { System.err.println("错误输出流"); Map<String,String> info = System.getenv(); for(String key : info.keySet()){ System.out.println(key+"="+System.getenv(key)); } } }
|
Runtime[了解]
含义
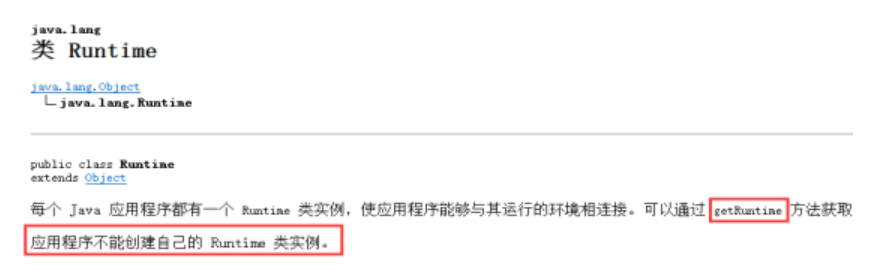
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class RuntimeDemo { public static void main(String[] args) { Runtime runtime = Runtime.getRuntime(); try { runtime.exec("shutdown -a"); } catch (IOException e) { e.printStackTrace(); } try { runtime.exec("mspaint"); } catch (IOException e) { e.printStackTrace(); } } }
|
BigDecimal
含义
用来表示任意精度的小数,常用在金额、高精度仪器
注意点
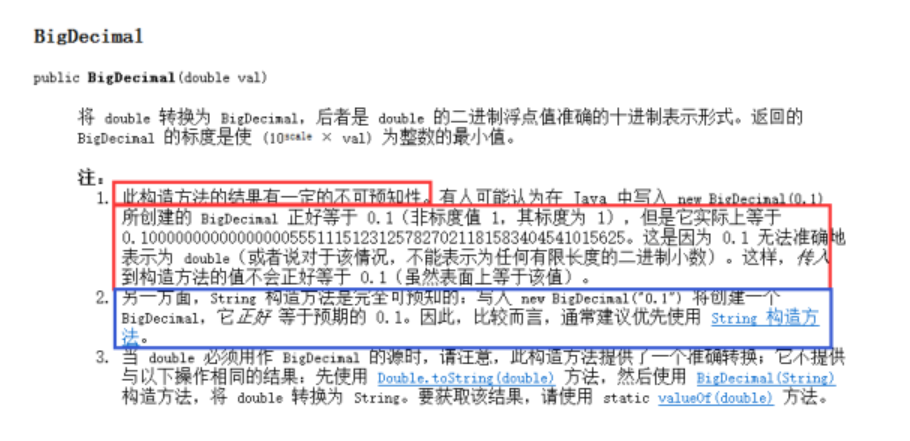
常用方法
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| public class BigDecimalDemo { public static void main(String[] args) { float data1 = 0.123456789f; float result = data1*0.1f; System.out.println(data1); System.out.println(result); BigDecimal one = new BigDecimal("0.123456789"); BigDecimal two = new BigDecimal("0.001"); BigDecimal three = one.add(two); System.out.println(three.toString()); three = one.subtract(two); three = one.divide(two); System.out.println(three.toString()); three = one.multiply(two); System.out.println(three.toString()); System.out.println(three.setScale(7, BigDecimal.ROUND_HALF_UP).toString()); } }
|
BigInteger
含义
无限大的整数
案例
TODO
含义
数字格式化
案例
TODO
集合
含义
容器,位于java.util包下面的类
数组特点
- 大小固定[不会自动扩容]
- 可以存基本数据、引用数据类型
集合特点
示意图
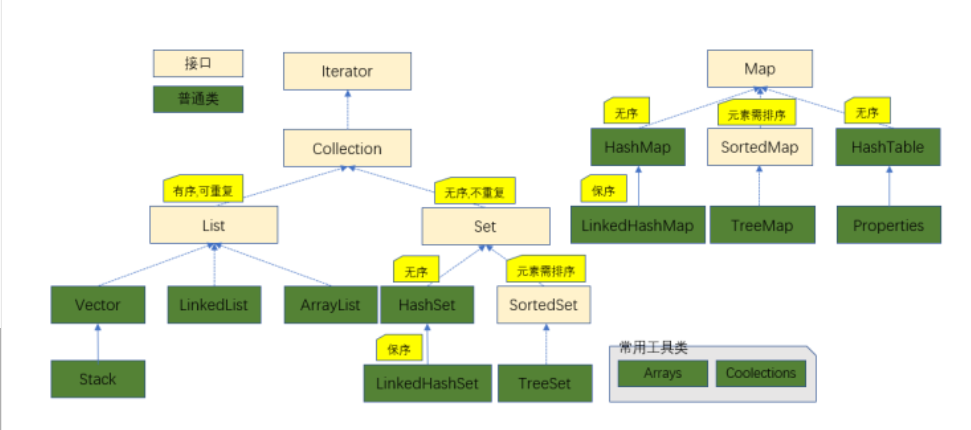
ArrayList
含义
是动态变长的数组
底层
是动态变长的数组
体系结构
常用方法
- isEmpty
- size
- add
- remove
- set
- contains
- get
- clear
- toArray
- …
案例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
| public class ArrayListDemo { public static void main(String[] args) { ArrayList data = new ArrayList(); data.add(22); data.add(33); data.add(0, 11); System.out.println(data.isEmpty()); System.out.println(data.size()); data.set(0,44); data.set(0,11); System.out.println(Arrays.toString(data.toArray())); System.out.println(data.contains(11)); data.remove(Integer.valueOf(11)); System.out.println(data.get(data.size()-1)); System.out.println(data); data.clear(); System.out.println(data); } }
|
String的equals 跟equalsIgnoreCase区别?
1、使用equals( )方法比较两个字符串是否相等。它具有如下的一般形式:
boolean equals(Object str)
这里str是一个用来与调用字符串(String)对象做比较的字符串(String)对象。如果两个字符串具有相同的字符和长度,它返回true,否则返回false。这种比较是区分大小写的。
2、为了执行忽略大小写的比较,可以调用equalsIgnoreCase( )方法。当比较两个字符串时,它会认为A-Z和a-z是一样的。其一般形式如下:
boolean equalsIgnoreCase(String str)
这里,str是一个用来与调用字符串(String)对象做比较的字符串(String)对象。如果两个字符串具有相同的字符和长度,它也返回true,否则返回false。